Collision detection in Unity is one of the most confusing aspects of Unity scripting for the aspiring game developer. No matter how many times you try to understand it, there's always a case where your methods aren't called and you have no idea why.
Fear not, choose the options that come closest to your situation and see the probable reason for your OnCollision
... or OnTrigger
... methods to not be called and fix it.
It will be trigger when the player approaches the obstacle, which I am starting to suspect is an enemy, but you will take actions in the script of the Player. Then you can keep the trigger for the raycast in the obstacle as is trigger, and you will know that the ontriggerenter in the obstacle script will be triggered when something hit the. Next make sure that your player collider has the 'Is Trigger' option selected in the Unity inspector. Next try using col.gameObject.CompareTag('Enemy') instead of: col.tag 'Enemy' in your if statement. Check out the Unity documentation as well on Colliders: Unity Collider documentation Unity Component.CompareTag method with example. Unity is the ultimate game development platform. Use Unity to build high-quality 3D and 2D games, deploy them across mobile, desktop, VR/AR, consoles or the Web, and connect with loyal and enthusiastic players and customers. Unity c# on trigger enter with specific gameobject. Csharp by IncineroarIron on Aug 06 2020 Donate. 1 void on trigger enter unity. Unity c# on trigger enter with specific gameobject. Csharp by IncineroarIron on Aug 06 2020 Donate. 1 void on trigger enter unity. Csharp by Beautiful Beetle on Dec 18 2020 Donate. Source: docs.unity3d.com. Add a Grepper Answer. C# answers related to “void on trigger enter unity”.
These options should cover both 2D and 3D, just remember that the 2D version has a 2D
appended at the end of both the method name and its parameter (e.g. OnTriggerEnter(Collider)
becomes OnTriggerEnter2D(Collider2D)
).
- Is it a trigger?
- Does at least one of the objects have Rigidbody attached?ARigidbody is necessary for at least one of the objects if you want to receive the
OnTriggerEnter/OnTriggerStay/OnTriggerExit
messages, even if the collider is a trigger. - One or both objects have a Rigidbody but it's still not working?Check the method's signature. The trigger methods (
OnTriggerEnter/OnTriggerStay/OnTriggerExit
) receive aCollider
argument, not aCollision
, this is a really common issue.- You checked the signature, but the methods are still not being called?Double check your colliders usingPhysics Debug Visualization.
Also, if this project is not entirely done by you, there's a chance that the objects involved in the collision are in layers configured to ignore collisions. Please see the unity official documentation on layer based collision.
- You checked the signature, but the methods are still not being called?
- Does it work sometimes?This might be happening because one or both objects are too fast for the discrete collision detection mode.
- Only one of the objects are moving fast.You need to set the fast-moving object collision detection mode to Dynamic. Note that you shouldn't default to setting everything to Dynamic (or Continuous Dynamic), this increases the CPU usage considerably, only use if necessary.
- Both objects are moving fast.If both are fast-moving objects you need to set the collision detection mode to Continuous Dynamic in the object you want to listen for the trigger event. The other object must be set to Dynamic or Continuous Dynamic.
Note that you shouldn't default to setting everything to Dynamic, this increases the CPU usage considerably, only use if necessary.
- Only one of the objects are moving fast.
- Does at least one of the objects have Rigidbody attached?
- Is it a regular collision?
- Does at least one of the objects have a non-kinematic Rigidbody attached?ARigidbody is necessary for at least one of the objects if you want to receive the
OnCollisionEnter/OnCollisionStay/OnCollisionExit
messages. - One or both objects have a non-kinematic Rigidbody but it's still not working?Check the method's signature. The collision methods (
OnCollisionEnter/OnCollisionStay/OnCollisionExit
) receive aCollision
argument, not aCollider
, this is a really common issue.- You checked the signature, but the methods are still not being called?Double check your colliders usingPhysics Debug Visualization.
Also, if this project is not entirely done by you, there's a chance that the objects involved in the collision are in layers configured to ignore collisions. Please see the unity official documentation on that.
- You checked the signature, but the methods are still not being called?
- Does it work sometimes?This might be happening because one or both objects are too fast for the discrete collision detection mode.
- Only one of the objects are moving fast.You need to set the fast-moving object collision detection mode to Dynamic. Note that you shouldn't default to setting everything to Dynamic (or Continuous Dynamic), this increases the CPU usage considerably, only use if necessary.
- Both objects are moving fast.If both are fast-moving objects you need to set the collision detection mode to Continuous Dynamic in the object you want to listen for the collision event. The other object must be set to Dynamic or Continuous Dynamic.
Note that you shouldn't default to setting everything to Dynamic, this increases the CPU usage considerably, only use if necessary.
- Only one of the objects are moving fast.
- Does at least one of the objects have a non-kinematic Rigidbody attached?
Additional Tips
You should not move static objects since this will make the physics engine recalculate the whole world. If something should move but shouldn't be affected by physics forces add a Rigidbody to it and make it kinematic.
Avoid using mesh colliders even if it has the same shape as of a primitive collider, the method of geometric intersection is completely different and the primitive colliders are much way faster.
If you are controlling an object by its transform, you must attach a Rigidbody in order to listen for the collision or trigger events, or in exact terms, for the methods OnTriggerEnter
, OnCollisionEnter
, etc to be called.
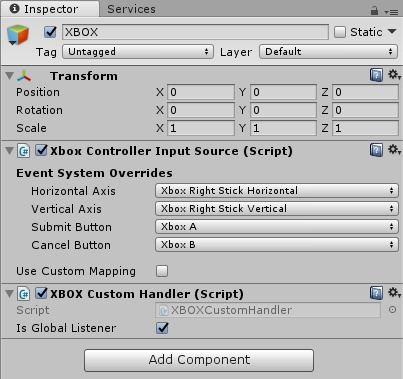
Kinematic Rigidbodies won't listen for collisions, but they can listen for trigger events, if marked as trigger.
For collision detection, what difference does it make to have a trigger collider?
Trigger colliders are less restrictive. Their rigidbodies can be kinematic or not, it doesn't matter, in either case the trigger message is going to be sent (OnTriggerEnter/OnTriggerStay/OnTriggerExit
methods will be called). If there's no Rigidbody attached they won't receive trigger messages.
Regular collisions using non-trigger colliders require that at least one of the involved objects have a non-kinematic Rigidbody attached.
'That's too complicated, how can I actually learn it?'
Right, you have fixed your problem but you're still wondering if you'll need to always reference a guide like this when dealing with collisions. Be cool because the answer is no, once you memorize the general gist of how this works you'll get it.
In general, you should start by adding rigidbodies to every object that you want to be able to listen for collisions. It's not necessary and not recommended to add a Rigidbody to a completely static object, a collider alone is sufficient and enough. If you need an object to listen for a collision but doesn't want it governed by the physics engine simulation, you mark it as kinematic.
'Nope, this guide is a farce, my collisions are still not working...'
I'm really sorry if you still aren't able to fix your collisions, my last attempt to help you is recommending you to read the official documentation on Colliders. It's really good and one of the base sources of this article.
Glossary
Unity Collider Is Trigger

Unity Is Trigger Collision
According to the official documentation kinematic rigidbodies should be used for colliders that may move or be enabled/disabled occasionally but that should otherwise behave like static colliders. This is good recommendation indeed since moving static objects is really CPU intensive and should be avoided.
Let's talk!
Haven't fixed your issue but still think I can help? Reach me on twitter @juliomarcos (I'm available for DMs). We can chat about other game development stuff too!
Main References of the article
Unity uses colliders to detect hits between different objects in the scene. If you have 2 cubes in the scene and you move one of top of the other nothing will happen. If instead you add a collider to both of them they will push each other around instead of stacking. Using a proper collider is a good advice for performance. It won’t matter if your scene is small and only has a few gameObjects, but once they start increasing the choice of a more computationally heavy collider will hit your performance hard. On the other hand the choice of a poor collider might make your collision detection less precise or even miss the collision entirely.
You can also receive a callback on collisions so that for example in a shooter you can decrease the HP of the hit target.
When you use a collider you have the choice of setting it as a trigger. If you do the collider will no longer react to other colliders, but instead it will act as an event so that you can react to it from code.
An example would be for powerups: I want to know when the player steps on a start so that I can add the power up to the player character. Using a trigger I can implement the function “OnTriggerEnter” or “OnTriggerEnter2D” in case of 2D colliders.
For testing purposes I wanted to receive a callback in both cases, simply add the following script to the gameObject you want to track. Create a file called TestCollision2D.cs and paste:
